OpenAI has significantly advanced its API capabilities, transitioning from the initial function_calls
method to the more sophisticated and comprehensive tool_calls
system. This update not only enhances how developers utilize Large Language Models (LLMs) but also introduces the ability to perform parallel tool calls, broadening the scope and efficiency of AI interactions. Let's delve into the distinctions between these methods and explore the new features introduced with tool_calls
.
Initial Approach: Function Calls
Initially, OpenAI utilized function_calls
, a method allowing developers to define specific tasks for the AI to execute. These tasks were structured in JSON format, detailing the function name, its parameters, and other relevant information. Here’s an example of how a function was defined:
1function_get_current_weather = {
2 "name": "get_current_weather",
3 "description": "Get the current weather in a given location",
4 "parameters": {
5 "type": "object",
6 "properties": {
7 "location": {
8 "type": "string",
9 "description": "The city and state, e.g. San Francisco, CA",
10 },
11 "unit": {"type": "string", "enum": ["celsius", "fahrenheit"]},
12 },
13 "required": ["location"],
14 },
15}
Example code to invoke a chat completion with functions:
1import json
2from openai import OpenAI
3
4client = OpenAI()
5
6response = client.chat.completions.create(
7 model="gpt-3.5-turbo-0613",
8 messages=[{"role": "user", "content": "What is the weather like in Boston today?"}],
9 functions=[function_get_current_weather],
10 function_call="auto",
11)
Using function_calls
, developers could directly interact with the AI to perform functions, but this approach had limitations, especially in handling parallel function calls. For instance, within a single chat completion request, it is not feasible to query multiple stock prices simultaneously, as only one tool function can be called at a time. This method is now marked as deprecated on OpenAI's official website.
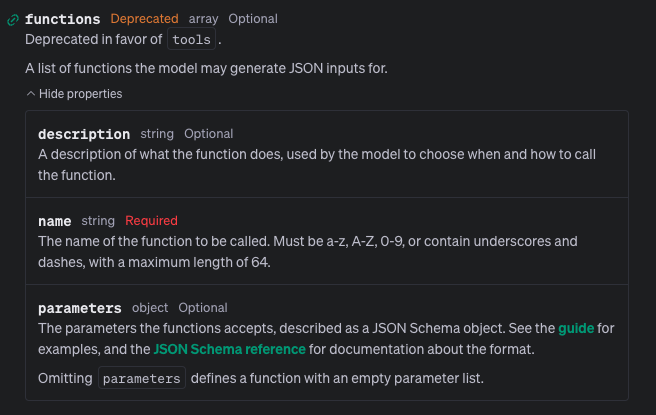
Update to Tool Calls
With the introduction of tool_calls
, OpenAI provided a more robust framework enabling more complex integrations and interactions. This update significantly enhances the model's utility by allowing parallel function calls and potentially integrating a broader range of tool types in the future.
Enhanced Features with Tool Calls
- Parallel Function Calling: This feature allows the model to execute multiple function calls simultaneously. For instance, the model can query the weather in three different locations at once. Each function call is managed independently within the
tool_calls
array, and responses are handled in parallel, reducing round trips and wait times. - Integration Flexibility: The structure of
tool_calls
is designed to support a wide variety of tools, not limited to functions. This design allows for future expansions and integrations of different tool types, enhancing the model's adaptability and application scope.
Here’s how you might use tool_calls
in practice:
1function_sum = {
2 "name": "add_two_number",
3 "description": "Add two number and return sum",
4 "parameters": {
5 "type": "object",
6 "properties": {
7 "a": {"type": "number", "description": "First number."},
8 "b": {"type": "number", "description": "Second number."},
9 },
10 "required": ["a", "b"],
11 },
12}
13
14response = client.chat.completions.create(
15 model="gpt-3.5-turbo-0613",
16 messages=[
17 {
18 "role": "user",
19 "content": "What is the weather like in Boston today? What is the result of 5698+21393?.",
20 }
21 ],
22 tools=[
23 {"type": "function", "function": function_get_current_weather},
24 {"type": "function", "function": function_sum},
25 ],
26 tool_choice="auto",
27)
28
29print(response)
In this example, the AI simultaneously processes a weather query and a mathematical operation, demonstrating the efficiency and power of parallel tool calls.
1ChatCompletion(id='chatcmpl-9OFYzBRLGQ2mQk9OnYYZiqNivIykW', choices=[Choice(finish_reason='tool_calls', index=0, logprobs=None, message=ChatCompletionMessage(content=None, role='assistant', function_call=None, tool_calls=[ChatCompletionMessageToolCall(id='call_6ysTzYj82dlRdwT4JZ1FaYw6', function=Function(arguments='{"location": "Boston", "unit": "celsius"}', name='get_current_weather'), type='function'), ChatCompletionMessageToolCall(id='call_OZBbeas822FBhmo952urTkJg', function=Function(arguments='{"location": "New York", "unit": "celsius"}', name='get_current_weather'), type='function')]))], created=1715566725, model='gpt-3.5-turbo-0125', object='chat.completion', system_fingerprint=None, usage=CompletionUsage(completion_tokens=56, prompt_tokens=87, total_tokens=143))
The transition from function_calls
to tool_calls
marks a significant improvement in how developers can leverage OpenAI's API to build more dynamic, efficient, and complex applications. This evolution not only increases the functionality but also prepares the platform for future enhancements and broader tool integrations.
TaskingAI's Unified Tool Interface
TaskingAI simplifies the integration and management of AI tools by offering a unified tool invocation interface. This means that regardless of the specific foundational model (e.g., OpenAI, Claude, Gemini, Mistral), you can use the same API format to invoke tools and functions. This uniformity facilitates seamless migration and integration of your tools across different AI models supported by TaskingAI.

TaskingAI offers a versatile suite of tools to enhance AI capabilities:
- Retrieval Tools: Allow AI to access and utilize large data sources for Retrieval-Augmented Generation, ideal for data-intensive tasks.
- Plugin Tools: Pre-configured and maintained by TaskingAI, including functionalities like search engines and calculators for easy integration.
- Action Tools: Facilitate interactions with web APIs, enabling AI to perform tasks such as booking appointments or sending emails through direct API calls.
- Function Tools: Customizable tools managed by clients, tailored to meet specific business needs with unique functionalities not covered by standard tools.
Once you've created an assistant with code or the UI console, you can easily programmatically interact with it.
Stateless Agent Invocation
1import taskingai
2
3ASSISTANT_ID = "YOUR_ASSISTANT_ID"
4
5# stateless agent invocation
6chat_completion_result = taskingai.inference.chat_completion(
7 model_id=ASSISTANT_ID,
8 messages=[
9 UserMessage("What is the weather like in Boston today?"),
10 ],
11 functions=[] # custom functions that fit OpenAI format
12)
Stateful Agent Invocation
1import taskingai
2
3ASSISTANT_ID = "YOUR_ASSISTANT_ID"
4
5# Create a new chat session
6chat = taskingai.assistant.create_chat(
7 assistant_id=ASSISTANT_ID,
8)
9
10# Send user query
11taskingai.assistant.create_message(
12 assistant_id=chat.assistant_id,
13 chat_id=chat.chat_id,
14 text="Calculate the distance between Earth and Mars.",
15)
16
17# Generate the assistant's response
18response = taskingai.assistant.generate_message(
19 assistant_id=chat.assistant_id,
20 chat_id=chat.chat_id,
21)
22
23print(response.content.text) # Display the assistant's response
Conclusion
With TaskingAI's diverse tool types, developers can build highly interactive and intelligent AI systems capable of handling complex queries and tasks. Whether it’s pulling data, performing calculations, interacting with web services, or executing custom functions, TaskingAI provides a unified and flexible interface to integrate a wide range of functionalities seamlessly.